Swiftui navigationbar toolbar toolbaritem placement toolbaritemplacement Reading time: 5 min This recipe is a cheatsheet for various ToolbarItemPlacement values and combinations on iOS. SwiftUI Cheat Sheet. Testing SwiftUI. Adding shadow and corner radius to a View. Strange behavior depending on View background color. Sample iOS project built by SwiftUI + MVVM and Combine framework using GitHub API. Rough attempt at creating a container. SimpleBoilerplates/SwiftUI-Cheat-Sheet is an open source project licensed under MIT License which is an OSI approved license. Labels are a much-needed addition in the latest SwiftUI iteration. They let you set icons alongside text with the following line of code. Label ('SwiftUI CheatSheet 2.0', systemImage: 'up.icloud') It's possible to set URL, upon clicking which will redirect to browser. SwiftUI is a gateway app development framework that has become one of the best ways for fledgling developers to get iOS apps off the ground without having to become a coding expert overnight. SwiftUI For Dummies makes that process even faster, providing a friendly introduction to the SwiftUI and Swift programming language and helping you feel.
Reading time: 5 min
This recipe is a cheatsheet for various ToolbarItemPlacement
values and combinations on iOS. This is useful because:
- The namings of the placement values don't necessarily clearly depict where will a
ToolbarItem
end up. - Some values don't play well with each other. E.g,
.primaryAction
will hide.confirmationAction
if it's placed above it, but not if placed below. - Some positions change if
TitleDisplayMode
is.inline
.
First we'll quickly introduce the toolbar
modifier, and then we'll look at how do various item placements and their combinations look on iOS.
TL;DR Here are the links to the bottom bar summary and navigation bar summary.
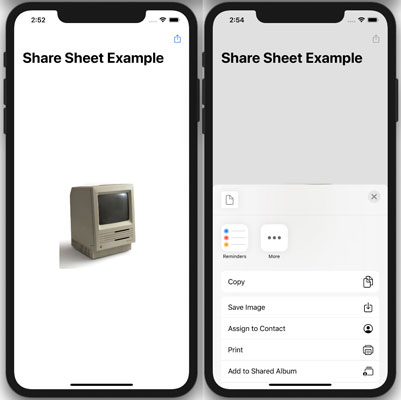
Intro to Toolbars
iOS 14 introduced the toolbar
modifier, allowing you to add ToolbarItem
s to either the toolbar (bottom bar) or the navigation bar. Note that this only works for views that are embedded in a NavigationView (either directly, or become via the navigation stack).
Here's a simple example that adds a few buttons around:
Note: The example uses the blueNavigation
modifier from this recipe on Navigation Bar Styling.
What happens here is:
- Views that use
toolbar
must be embedded inNavigationView
. Alternatively, the view from which you navigated to viaNavigationLink
should be. - Use the same
toolbar
modifier for all items, regardless of if they're in the navigation bar or bottom bar. - Use the
ToolbarItem
wrapper to add items to the bar and specify the position withplacement:
. - Add a regular
Button
with a title and empty action.
The result looks like this. You can see two buttons, one added to the navigation bar and one to the bottom toolbar:
Okay, time to dive into all the placements and their combos!
ToolbarItemPlacement and its values
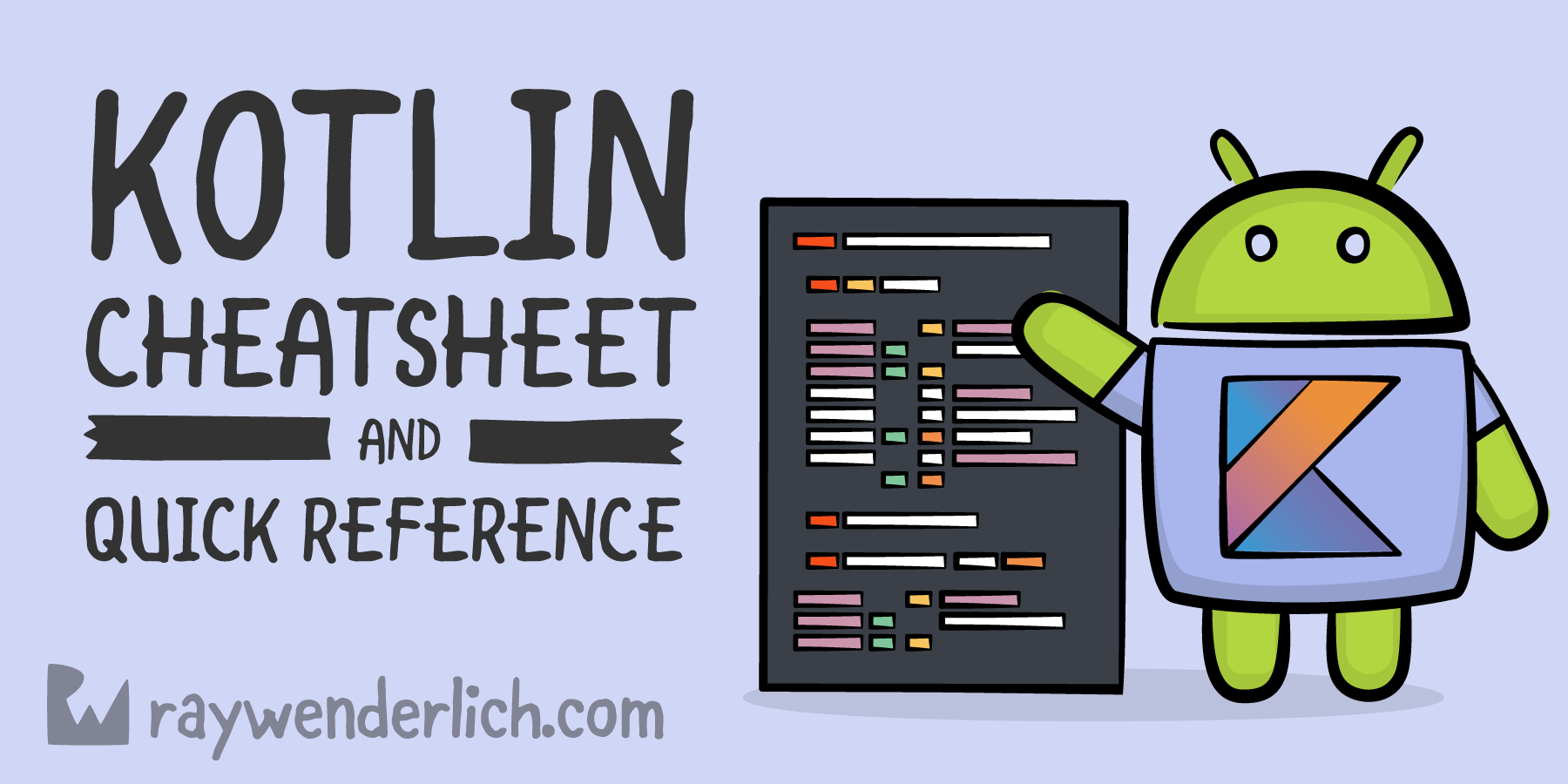
The ToolbarItemPlacement
is a struct with a bunch of static constants representing different toolbar placements (you can think of it as an enum, even though it's not implemented that way). Here are all the supported placement values:
automatic
principal
navigation
primaryAction
status
confirmationAction
cancellationAction
destructiveAction
navigationBarLeading
navigationBarTrailing
bottomBar
We'll look at the placements that affect the bottom space (bottom toolbar) and the top space (navigation bar) separately, as they don't affect each other.
Fot the sake of brevity, example will just show the code inside the toolbar
modifier block.
Bottom bar placements
The bottom bar placements are status
and bottomBar
.
status
is placed in the center of the toolbar.bottomBar
starts from the leading edge of the toolbar.
If you add another bottomBar
to the list:
it goes to the trailing edge:
If you keep adding more bottomBar
items:
Swiftui Sheet Size
they'll keep lining up at the trailing edge:
Multiple status
items:
Swiftui Cheat Sheet Pdf
are just sequentially lined up in the center (if possible):
Navigation bar placements
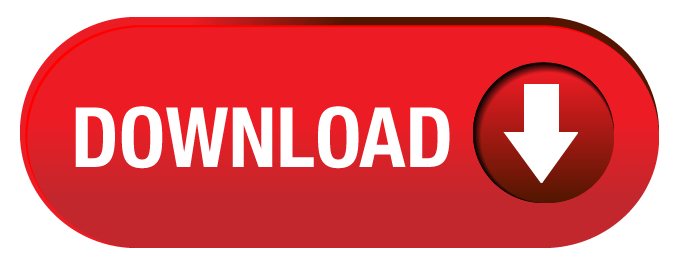